What's AndEngine
AndEngine is a 2d Engine OpenGL for the Android platform.There is the AndEngine Core Terminology taken directly from the AndEngine forums.
- BaseGameActivity: The BaseGameActivity is the root of a game, that contains an Engine and manages to create a SurfaceView the contents of the Engine will be drawn into. There is always exactly one Engine for one BaseGameActivity. You can proceed from one BaseGameActivity to another using common Android mechanisms.
- Engine: The Engine make the game proceed in small discrete steps of time. The Engine manages to synchronize a periodic drawing and updating of the Scene, which contains all the content that your game is currently handling actively. There usually is one Scene per Engine, except for the SplitScreenEngines.
- IResolutionPolicy: An implementation of the IResolutionPolicy interface is part of the EngineOptions. It tells AndEngine how to deal with the different screen-sizes of different devices. I.e. RatioResolutionPolicy will maximize the SurfaceView to the limiting size of the screen, while keeping a specific ratio. That means objects won't be distorted while the SurfaceView has the maximum size possible.
- Camera: A Camera defines the rectangle of the scene that is drawn on the screen, as not the whole scene is visible all the time. Usually there is one Camera per Scene, except for the SplitScreenEngines. There are subclasses that allow zooming and smooth position changes of the Camera.
- Scene: The Scene class is the root container for all objects to be drawn on the screen. A Scene has a specific amount of Layers, which themselves can contain a (fixed or dynamic) amount of Entities. There are subclasses, like the CameraScene/HUD/MenuScene that are drawing themselves to the same position of the Scene no matter where the camera is positioned to.
- Entity: An Entitiy is an object that can be drawn, like Sprites, Rectangles, Text or Lines. An Entity has a position/rotation/scale/color/etc...
- Texture: A Texture is a 'image' in the memory of the graphics chip. On Android the width and height of a Texture has to be a power of 2. Therefore AndEngine assembles a Texture from a couple of ITextureSources, so the space can be used better.
- ITextureSource: An implmentation of the ITextureSource-interface like AssetTextureSource manages to load an image onto a specific position in the Texture.
- TextureRegion: A TextureRegion defines a rectangle on the Texture. A TextureRegion is used by Sprites to let the system know what part of the big Texture the Sprite is showing.
- PhysicsConnector: A PhysicsConnector manages to update the AndEngine-Shapes (like Rectangles/Sprites/etc...) when their physics representations "bodies" change. Once using Physics (and a PhysicsConnector) with an AndEngine-Shape you'd disable the Physics calculated by AndEngine itself, by calling setUpdatePhysics(false) to the Shape. Changes made to the AndEngine-Shape are not reflected in the Physics - you have to call the methods on the Body object you have used to create the PhysicsConnector.
Getting AndEngine
To get Andengine, you can download sources from http://www.andengine.org or you can download a jar file. I'm using the jar file option.
First download this file and keep it in your computer.
Eclipse project Creation
Start new Project with File -> New Project.
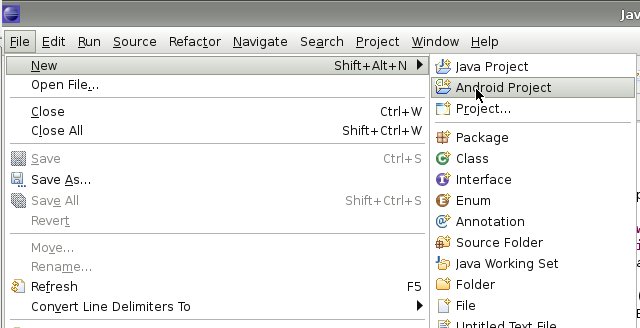
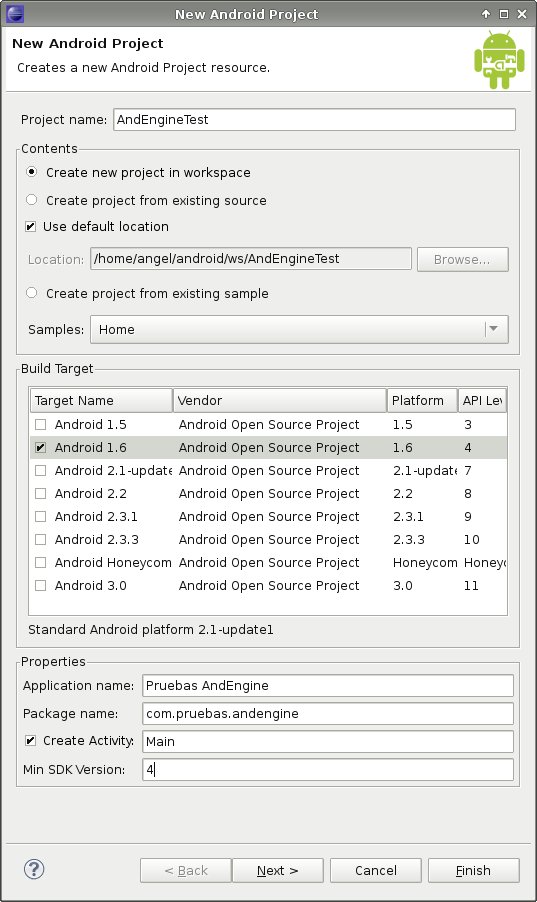
Next, Finish.. Now we create a lib folder under the root directory of our project.
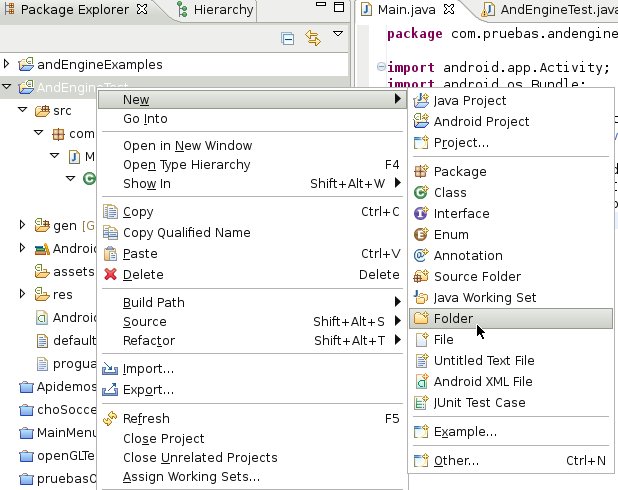
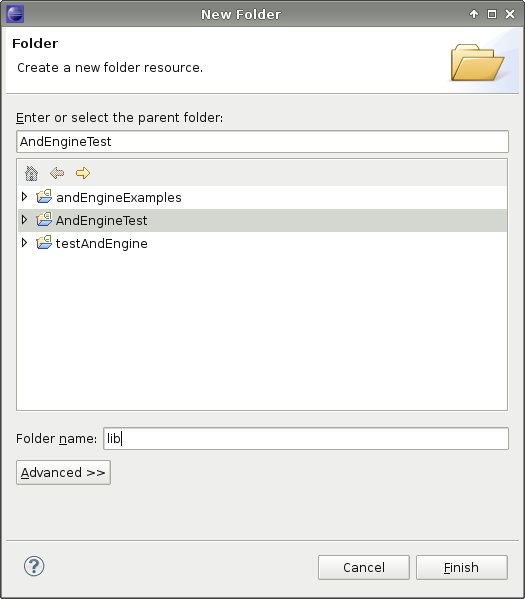
Now drag the jar file previously downloaded to the lib folder in Eclipse. It looks like this..
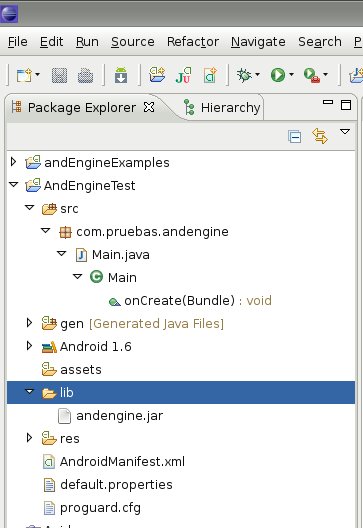
Now Right Button on the andengine.jar file and Build Path -> Add to Build Path.
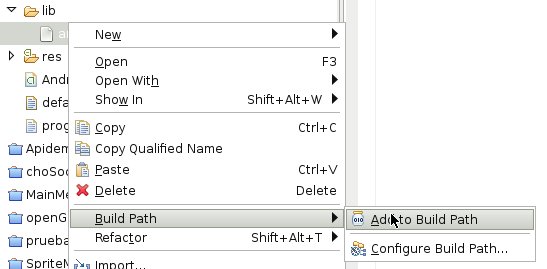
Now the go to Main.java file and change Activity to BaseGameActivity. delete the default constructor, organize imports, and implement the BaseGameActivity methods. Now it should look like this code below.
Main.java
package com.pruebas.andengine;
import org.anddev.andengine.engine.Engine;
import org.anddev.andengine.entity.scene.Scene;
import org.anddev.andengine.ui.activity.BaseGameActivity;
import android.os.Bundle;
public class Main extends BaseGameActivity {
@Override
public void onLoadComplete() {
// TODO Auto-generated method stub
}
@Override
public Engine onLoadEngine() {
// TODO Auto-generated method stub
return null;
}
@Override
public void onLoadResources() {
// TODO Auto-generated method stub
}
@Override
public Scene onLoadScene() {
// TODO Auto-generated method stub
return null;
}
}
Parsed in 0.072 seconds at 8.45 KB/s, using GeSHi 1.0.8.10
Now let's change a little the file to make it clear.
Main.java
import org.anddev.andengine.engine.Engine;
import org.anddev.andengine.entity.scene.Scene;
import org.anddev.andengine.ui.activity.BaseGameActivity;
public class Main extends BaseGameActivity {
// ===========================================================
// Constants
// ===========================================================
// ===========================================================
// Fields
// ===========================================================
// ===========================================================
// Constructors
// ===========================================================
// ===========================================================
// Getter & Setter
// ===========================================================
// ===========================================================
// Methods for/from SuperClass/Interfaces
// ===========================================================
@Override
public void onLoadComplete() {
// TODO Auto-generated method stub
}
@Override
public Engine onLoadEngine() {
// TODO Auto-generated method stub
return null;
}
@Override
public void onLoadResources() {
// TODO Auto-generated method stub
}
@Override
public Scene onLoadScene() {
// TODO Auto-generated method stub
return null;
}
// ===========================================================
// Methods
// ===========================================================
// ===========================================================
// Inner and Anonymous Classes
// ===========================================================
}
Parsed in 0.092 seconds at 17.27 KB/s, using GeSHi 1.0.8.10
Let's Start
Now we need a camera object to the proper view of the game. Let's create variables to define the screen resolution we are going to work for and a TAG variable for debugging purposes.
// ===========================================================
// Constants
// ===========================================================
static final int CAMERA_WIDTH = 480;
static final int CAMERA_HEIGHT = 320;
private static final String TAG = "AndEngineTest";
// ===========================================================
// Fields
// ===========================================================
private ZoomCamera mCamera;
Parsed in 0.076 seconds at 5.72 KB/s, using GeSHi 1.0.8.10
Now let's write some code on the on the onLoadEngine() method.
public Engine onLoadEngine() {
this.mCamera = new ZoomCamera(0, 0, CAMERA_WIDTH, CAMERA_HEIGHT);
return new Engine(new EngineOptions(true, ScreenOrientation.LANDSCAPE, new RatioResolutionPolicy(CAMERA_WIDTH, CAMERA_HEIGHT), this.mCamera));
}
Parsed in 0.084 seconds at 2.84 KB/s, using GeSHi 1.0.8.10
We have a camera object now. Let's implement the method onLoadScene() and put some background color.
@Override
public Scene onLoadScene() {
this.mEngine.registerUpdateHandler(new FPSLogger());
final Scene scene = new Scene(1);
scene.setBackground(new ColorBackground(0, 0, 0.8784f));
return scene;
}
Parsed in 0.076 seconds at 2.66 KB/s, using GeSHi 1.0.8.10
After organize imports and some organization...
import org.anddev.andengine.engine.Engine;
import org.anddev.andengine.engine.camera.ZoomCamera;
import org.anddev.andengine.engine.options.EngineOptions;
import org.anddev.andengine.engine.options.EngineOptions.ScreenOrientation;
import org.anddev.andengine.engine.options.resolutionpolicy.RatioResolutionPolicy;
import org.anddev.andengine.entity.scene.Scene;
import org.anddev.andengine.entity.scene.background.ColorBackground;
import org.anddev.andengine.entity.util.FPSLogger;
import org.anddev.andengine.ui.activity.BaseGameActivity;
public class Main extends BaseGameActivity {
// ===========================================================
// Constants
// ===========================================================
static final int CAMERA_WIDTH = 480;
static final int CAMERA_HEIGHT = 320;
private static final String TAG = "AndEngineTest";
// ===========================================================
// Fields
// ===========================================================
private ZoomCamera mCamera;
// ===========================================================
// Constructors
// ===========================================================
// ===========================================================
// Getter & Setter
// ===========================================================
// ===========================================================
// Methods for/from SuperClass/Interfaces
// ===========================================================
@Override
public void onLoadComplete() {
// TODO Auto-generated method stub
}
@Override
public Engine onLoadEngine() {
this.mCamera = new ZoomCamera(0, 0, CAMERA_WIDTH, CAMERA_HEIGHT);
return new Engine(new EngineOptions(true, ScreenOrientation.LANDSCAPE, new RatioResolutionPolicy(CAMERA_WIDTH, CAMERA_HEIGHT), this.mCamera));
}
@Override
public void onLoadResources() {
// TODO Auto-generated method stub
}
@Override
public Scene onLoadScene() {
this.mEngine.registerUpdateHandler(new FPSLogger());
final Scene scene = new Scene(1);
scene.setBackground(new ColorBackground(0, 0, 0.8784f));
return scene;
}
// ===========================================================
// Methods
// ===========================================================
// ===========================================================
// Inner and Anonymous Classes
// ===========================================================
}
Parsed in 0.093 seconds at 26.05 KB/s, using GeSHi 1.0.8.10
Let's start the application to see a Background color. In the Top menu "Run" Configurations
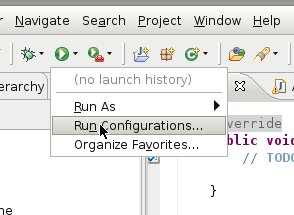
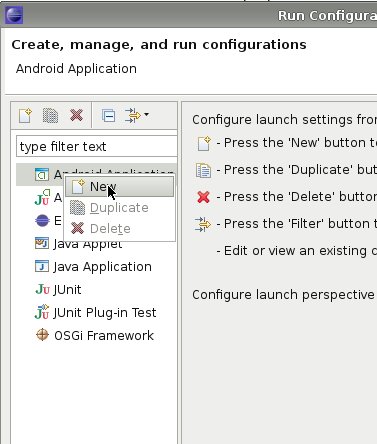
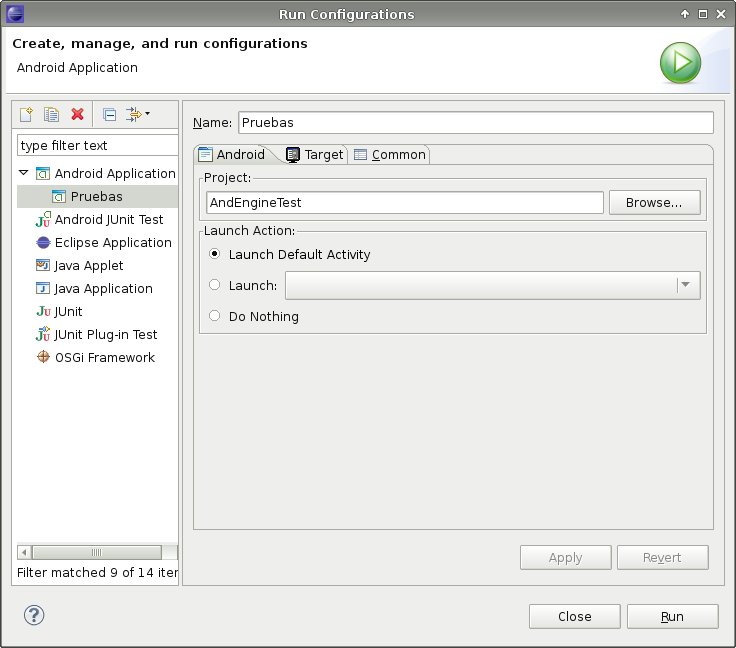
Apply -> Run and now we see in the terminal our first AndEngine application.
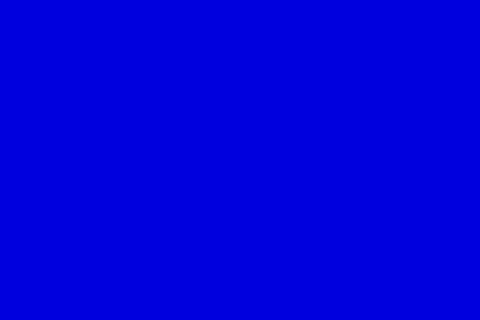
This is the end of the first lesson in the series. In the second tutorial, we are gonna paint some objects in the screen, and implement scrolling with TouchEvents.
Source Code
To get the source code: svn checkout http://ch-soccer.googlecode.com/svn/trunk/ tutorial-read-only -r 3
eclipse throwing error
ReplyDelete[2011-03-31 00:55:17 - AndEngineTest] Error generating final archive: Found duplicate file for APK: AndroidManifest.xml
Origin 1: C:\workspace\AndEngineTest\bin\resources.ap_
Origin 2: C:\workspace\AndEngineTest\lib\andengine.jar
jar file includes another androidmanifest.xml files
ReplyDeleteI'm uploading my andengine.jar to mediafire to ensure we are all using the same .jar.
ReplyDeleteThe link is -> http://www.mediafire.com/?sz7xjjohbk3vo6o
Great :D thanks
ReplyDeleteHey, thanks for the detailed examples.
ReplyDeleteIt seems that your donate button is broken. Could you check if it's ok? I'd love to donate couple of bucks for your efforts.
Do email me when you have fixed the button, so I wont forget :)
Getting error
ReplyDeleteError generating final archive: Found duplicate file for APK: AndroidManifest.xml
I'm seeing a deprecation error around line 61 for:
ReplyDeletefinal Scene scene = new Scene(1);
See http://www.andengine.org/forums/post17531.html for some relevant advice
I just used Scene() for the deprecated error.
ReplyDeleteThis comment has been removed by the author.
ReplyDeletepublic Engine onLoadEngine()
ReplyDelete{
this.mCamera = new ZoomCamera(0,0, CAMERA_WIDTH, CAMERA_HEIGHT);
return new Engine(new EngineOptions(true, ScreenOrientation.LANDSCAPE, new RatioResolutionPolicy(CAMERA_WIDTH, CAMERA_HEIGHT), this.mCamera));
}
Here, the error is
"The constructor Engine(EngineOptions) is undefined"
asdasd make sure you have imported the Engine. Add this line under your last import statement :)
ReplyDeleteimport org.anddev.andengine.engine.Engine;
This comment has been removed by the author.
ReplyDeleteI'm getting a java.lang.RuntimeException: Unable to instantiate activity ComponentInfo
ReplyDeleteAny ideas what i've done wrong?
Problem solved.
DeleteJust had to change the name of the folder from lib to libs :)